The type safe
serverless platform for
modern full-stack developers
Everything you need to build apps that scale up to millions of users.
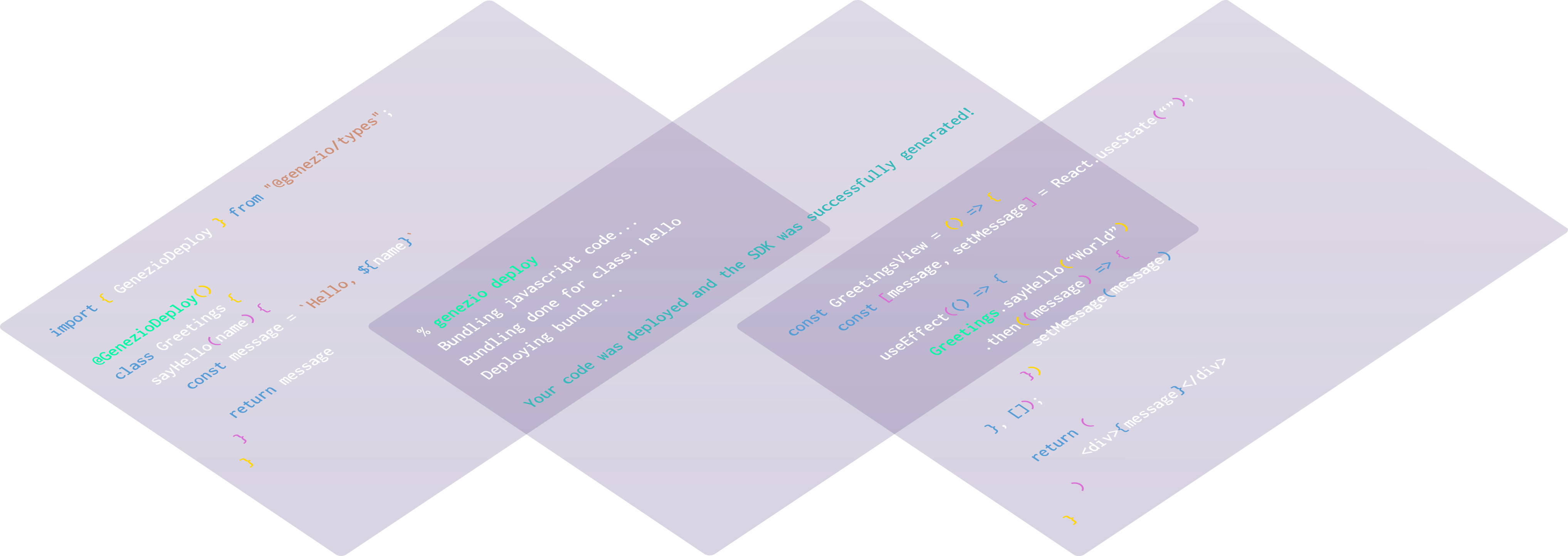
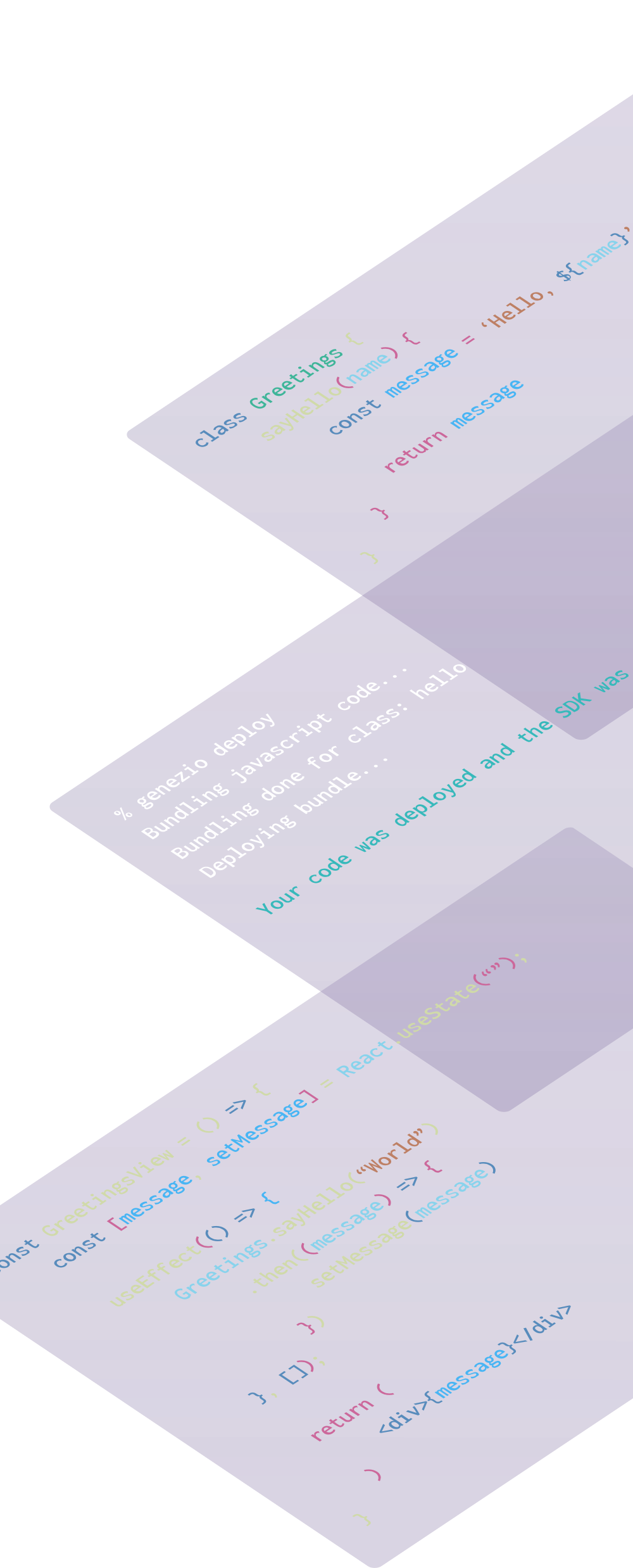

Hosting included
for all plans
USED AND TRUSTED AT
Your app, your code, your data.
If you are a full-stack developer who needs to build, deploy and maintain web, mobile or enterprise apps that scale automatically, and use type safe frontend-backend communication, then Genezio is for you. There are many solutions that are easy to use, but hide their magic and trap you in. With Genezio, you own all the code and the databases that we help you set up.
CAPABILITIES
Genezio in a nutshell
Type safe RPC backend breaks less often
- Full static type safety with auto-completion in your favorite editor. Dynamic type safety at runtime.
- Tested and production ready for TypeScript BE/FE. Beta support for GoLang, Kotlin and DART.
- Export the resulting SDK using dependency managers such as NPM Registry - private to your team or public for anybody to use.
Easy to use databases, queues, crons, auth...
- Databases - provisioned by us or you can bring your own. Table creation and CRUD boilerplate functions generated through LLM.
- Cron jobs - scheduled to be executed up to a minute granularity.
- User authentication module - works on any database.
- Email service - use it in your code to send emails to your users securely.
Host your app for free with auto-scaling
- Framework agnostic, works with React, Vue, Angular, Svelte, ExpressJS, Fastify, etc.
- Developer experience enhanced bundling - minimal package generated automatically.
- Deploy the backend on managed AWS or Genezio.Cloud. Frontend deployment with CDN.
- Multiple staging environments supported, production deployment as well as local development environment.
Dashboard with test-interface
Collaborate with your teammates
Automatic scaling
View and search live logs
Framework agnostic - React, Vue, Svelte, etc
Open source community
Show me the code
A more natural paradigm in software design, leading to less error prone parameter passing than REST APIs and auto completion in your favorite IDE. Here we define the "bye" method in the HelloWorldService backend class, and we call it from the frontend using the auto-generated SDK.
type Person = {
firstName: string;
lastName: string;
}
@GenezioDeploy()
class HelloWorldService {
hello(name: string): string {
return `Hello ${name}!`;
}
bye(person: Person): string {
return `Bye, ${person.firstName} ${person.lastName}!`;
}
}
import { HelloworldService, Person } from '@genezio-sdk/helloworld-app';
const hi = await HelloworldService.hello('John');
const person: Person = {firstName: 'John', lastName: 'Ronald'};
const bye = await HelloworldService.bye(person);
We parse the backend code you write and store the AST in a database. From there we generate client side stubs in any language. It's like tRPC but for any backend language to any frontend language.
Now supporting JavaSript/TypeScript, with support for DART/Flutter, Kotlin, Swift, GO, Python and others coming soon.
import { HelloworldService, Person } from '@genezio-sdk/helloworld-app';
async function main() {
const hi = await HelloworldService.hello('John');
const person: Person = {firstName: 'John', lastName: 'Ronald'};
const bye = await HelloworldService.bye(person);
}
import 'package:hello_world/sdk/hello_world_service.dart';
void main() async {
final hi = await HelloWorldService.hello('John');
final person = Person(firstName: 'John', lastName: 'Ronald');
final bye = await HelloWorldService.bye(person);
}
import sdk.HelloWorldService
import sdk.Person
suspend fun sayHi() {
val hi = HelloWorldService().hello("John")
val person = Person(firstName = "John", lastName = "Ronald")
val bye = HelloWorldService().bye(person)
}
Authenticate users securely with multiple login methods, such as Email/Password, Google, and more coming soon. Use PostgreSQL or MongoDB provisioned from the Genezio Dashboard, or bring your own database. No vendor lock-in; take control of your code at any time.
import { AuthService } from '@genezio/auth';
AuthService.get().setTokenAndRegion("1-xyz", "us-east-1")
const Signup = () => {
// rest of the code
const handleSignupSubmit = async (e) => {
// Use the auto generated EmailAuthService to register the user
const response = await AuthService.get().register(user.email, user.password, user.username)
if (response.success) { ... }
}
const handleLoginSubmit = async (e) => {
// Use the auto generated EmailAuthService to register the user
const response = await AuthService.get().login(user.email, user.password)
if (response.success) { ... }
}
const getUserInfo = async (e) => {
// Use the auto generated EmailAuthService to register the user
const userInfo = await AuthService.get().getUserInfo()
...
}
// rest of the code
}
import { GenezioDeploy, GnzContext, GnzAuth } from '@genezio/types';
@GenezioDeploy()
export class UserInfoService {
@GnzAuth
async getUserInfo(context: GnzContext): Promise<void> {
const userInfo = await UserModel.getUserInfo(context.user.id)
return userInfo
}
}
We offer Redis or Postgres through our partners. Or bring your own database and connect it to your backend like you normally would.
import { GenezioDeploy } from "@genezio/types";
import pg from 'pg'
const { Pool } = pg
@GenezioDeploy()
export class PostgresService {
pool = new Pool({
connectionString: process.env.NEON_POSTGRES_URL,
ssl: true,
});
async insertUser(name: string): Promise<string> {
await this.pool.query(
"CREATE TABLE IF NOT EXISTS users (id serial PRIMARY KEY,name VARCHAR(255))"
);
await this.pool.query("INSERT INTO users (name) VALUES ($1)", [name]);
const result = await this.pool.query("select * from users");
return JSON.stringify(result.rows);
}
}
import { GenezioDeploy } from '@genezio/types';
import { Redis } from '@upstash/redis'
@GenezioDeploy()
export class RedisService{
client = new Redis({
url: process.env.UPSTASH_REDIS_URL,
token: process.env.UPSTASH_REDIS_TOKEN,
})
async insertUser(name: string): Promise<string> {
await this.client.set('user', name);
const result = await this.client.get('user');
return JSON.stringify(result);
}
}
Manage and process large volumes of tasks and requests with ease and reliability. Extremely helpful when you need to do something asynchronously.
import { GenezioDeploy, GenezioMethod,
GenezioHttpRequest, GenezioHttpResponse } from "@genezio/types";
import axios from 'axios';
@GenezioDeploy()
export class QstashService{
async chatbot(): Promise<boolean> {
const url = `https://qstash.upstash.io/v2/publish/${process.env.QUEUE_WEBHOOK_URL}`;
const payload = {
"message": "I am your trusty chatbot! I'll help you process your tasks.",
};
const headers = {
Authorization: `Bearer ${process.env.QSTASH_TOKEN}`,
'Content-Type': 'application/json',
};
await axios.post(url, payload, { headers: headers })
return true
}
@GenezioMethod({ type: 'http' })
async sendReplyToChatApp(request: GenezioHttpRequest): Promise<GenezioHttpResponse> {
const message: string = request.body.message;
await this.#sendReplyToChatApp(message);
const response: GenezioHttpResponse = {
body: {"status":"ok"},
headers: { 'content-type': 'application/json' },
statusCode: '200',
};
return response;
}
}
Support for configurable cron jobs to automate your application.
import { GenezioDeploy } from '@genezio/types';
@GenezioDeploy()
export class OrderService {
// this method will run every day at midnight
@GenezioMethod({ type: "cron", cronString: '0 0 * * *' })
async checkForUnfinishedOrders() {
const unfinishedOrders = await this.orders.find({
where: {
status: 'unfinished'
}
});
await this.sendReminderEmails(orders);
}
}
Store and retrieve user-generated content, application settings or multimedia files.
We will support long running servers through genezio.deploy on AWS and we will provide real-time communication between the backend and the frontend.
Genezio offers multi-cloud support, including AWS and the new Genezio.Cloud that's still being tested. We're using AWS Lambda right now, and we're working to add long running servers. Plus, we provide support to deploy on your own AWS account.
% ls
client/ server/ genezio.yaml
% genezio deploy
Bundling your code...
Deploying your server...
The server was deployed and the SDK was successfully generated!
You can test it at https://app.genez.io/project/a1b2c3d4-e5f6-7890-g1h2-i3j4k5l6m7n8/a1b2c3d4-e5f6-7890-g1h2-i3j4k5l6m7n9
Deploying your frontend...
Frontend successfully deployed at https://happy-purple-capybara.app.genez.io
% ls
client/ server/ genezio.yaml
% genezio local
Bundling your code...
Starting your local server...
Your local server is running, and the SDK was successfully generated!
Test your code at http://localhost:8083/explore
Deploy to local, stage or production with one command.
% ls
client/ server/ genezio.yaml
% genezio deploy --stage dev
Bundling your code...
Deploying your server...
The server was deployed, and the SDK was successfully generated!
You can test it at https://app.genez.io/project/a1b2c3d4-e5f6-7890-g1h2-i3j4k5l6m7n8/a1b2c3d4-e5f6-7890-g1h2-i3j4
% ls
client/ server/ genezio.yaml
% genezio local
Bundling your code...
Starting your local server...
Your local server is running, and the SDK was successfully generated!
Test your code at http://localhost:8083/explore
Deploy to AWS CloudFront now. Working to integrate with Netlify.
% ls
client/ server/ genezio.yaml
% genezio deploy --frontend
Deploying your frontend...
Frontend successfully deployed at https://happy-purple-capybara.app.genez.io
Test parameters: Hello World function 30 HTTPS requests for each scenario Cached DNS records for functions domains to prevent additional time caused by DNS
Show me the code
EDUCATION
Our latest article
TESTIMONIALS
Don't take our word for it
Genezio has significantly improved my development workflow, both for my startup projects and at work. The platform enables me to quickly develop, test, and deploy new features. Its ease of integration allowed my team to offload work seamlessly, enhancing our productivity. Genezio’s efficient and user-friendly interface has made it a valuable tool in our development process.
FAQ
Your questions answered
- Flexibility: Genezio supports various programming languages, making it more versatile for developers with different tech stacks.
- Type Safety: Genezio provides a type safe interface, reducing errors and enhancing code quality.
- Full-Stack Support: You can connect Genezio with any frontend, whether it's for web, mobile, or desktop applications, and still maintain type safety.
- Productivity: Genezio offers a range of built-in services like authentication, cron jobs and database integration, making development faster and more efficient.
- Scalability: Genezio ensures auto-scalability for your applications, adapting to traffic demands effortlessly.