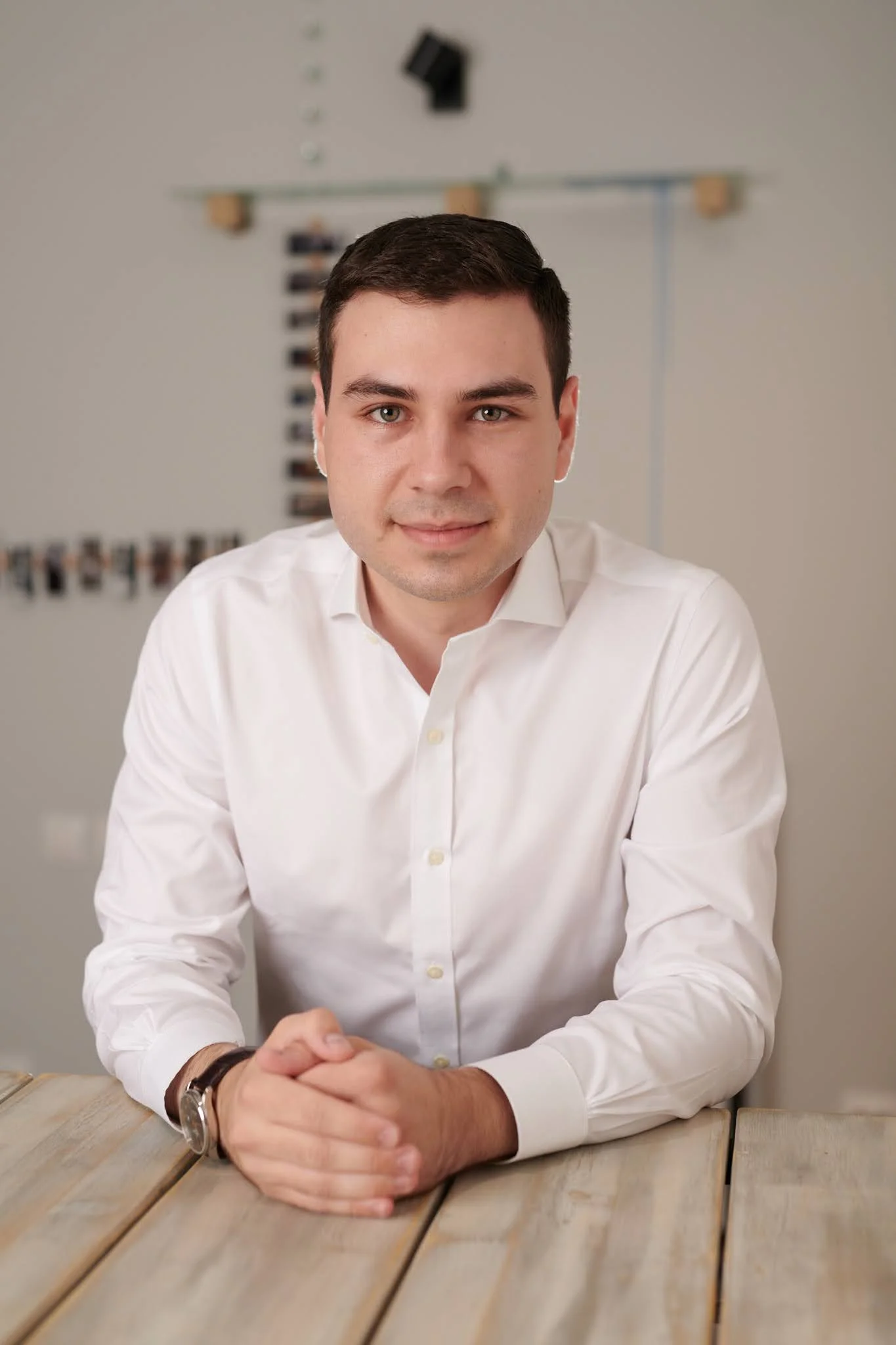
Radu Dumitrescu
Jul 06, 2023
In this tutorial, I will show you the steps of integrating a Mongo database into your genezio Getting Started project .
Following this tutorial, you will be able to:
- Create a free-tier MongoDB Atlas cluster
- Connect to the MongoDB cluster using a MongoDB client
- Integrate the Mongo database into your project using
mongoose
Any time you get stuck or have questions, please contact me on Discord or write me an email at radu@genez.io. I am more than happy to help you 😄
Contents
Introduction
- Why MongoDB Atlas?
Hands-on Tutorial
- Create a MongoDB Atlas account
- Create a free cluster
- Integrate your newly created cluster into the project
- Create a new DB connection into a class
Check your DB with MongoDB Compass
Insights
Pre-requisites
Have
npm
installed on your machineHave
mongoose
installed on your machineCreate a free genezio account here
Have
genezio
installed on your machine - you can install it withnpm install genezio -g
Introduction
Why MongoDB Atlas?
As a cloud database service, MongoDB Atlas offers seamless scalability, high availability, and robust security. You can focus on building innovative applications while leaving the complexities of database management behind thanks to its intuitive interface and seamless integration with major cloud providers.
Hands-on Tutorial
Create a MongoDB Atlas account
Go to MongoDB Cloud Atlas and create an account. Personally, I recommend creating an account using Google Sign up.
Create a free cluster
After you create your account, you will get to this wizard where you can create your cluster:
Here you have the following configurations:
- Select the free tier M0
- Select the cloud provider - we recommend choosing AWS
- Select the region of the cloud provider - this should be as close to you and your customers as possible
- Give your cluster a name
Click the ‘Create’ button.
After, you have to create a user with a strong password. Make sure to store it somewhere safe as we will need it later on.
Fill in the required information and click ‘Create User’.
Next, add an IP address to the whitelist. For testing purposes, you can add 0.0.0.0
- this means IPs are allowed. I highly discourage using this in a production environment.
Now click on ‘Add Entry’.
You should now see your dashboard. Click on ‘Connect’ in the cluster.
A pop-up with the MongoDB connection string will appear. Replace <password>
with your password from the previous step and copy it.
Integrate your newly created cluster into the project
Now you can go to your Getting Started project and open an IDE of your choice. I recommend VS Code.
In the ‘server’ folder, create a .env
file and add a line with the MONGO_DB_URI=<your_connection_string>
value. You can then use it all over your code using process.env.MONGO_DB_URI
.
Create a new DB connection into a class
Now that we have the connection string, you can integrate MongoDB into your classes.
First, you have to import mongoose
:
server/backendService.ts
import mongoose from "mongoose";
@GenezioDeploy()
export class TutorialClass {
constructor() {
this.#connect();
}
/**
* Private method used to connect to the DB.
*/
#connect() {
mongoose.connect(process.env.MONGO_DB_URI).catch((error) => {
console.log("Error connecting to the DB", error);
});
}
}
Into the class’s constructor, you need to establish the connection with the database. I recommend doing this in a separate private method as in the code shown above.
If you want your code to look cleaner, you can write the connection method in another file and just import and call it in your class.
After that, you can use all the functions provided by mongoose all over the functions from your class.
Check your DB with MongoDB Compass
MongoDB Compass is one of the most powerful database clients for MongoDB. You can download it from here .
I highly recommend using it for GUI queries to your database, and also for debugging your application.
After you install it, you can simply open it, add your MongoDB Connection String, and then you will have full admin access to your database.
Be careful when handling a database that is used in a production environment because you can delete things from there.
Insights
Database connection error
You might encounter an issue when connecting to your database. One of the main reasons is that your IP might not be allowed to access the cluster. To change this, go to your cluster configuration, and on the tab ‘Network Access’ add your IP or 0.0.0.0
to give full IP access to the database.
Serverless database access
With MongoDB Atlas, there are 2 main ways to query the database.
Persistent Connection
This is the most conventional method of establishing a connection to a database, but [IT] encounters certain issues in a serverless environment, particularly when the initial connection consumes a significant amount of time.
Data API
Mongo DB Atlas Data API offers a solution where you can directly access the database through an API, eliminating the requirement of establishing an initial connection and reducing serverless cold-start significantly.
🥳 Congratulations on acquiring your own database that you can use to work on your projects!
Article contents
Subscribe to our newsletter
Genezio is a serverless platform for building full-stack web and mobile applications in a scalable and cost-efficient way.
Related articles
More from Tutorials
How to Use Genezio with Express for Easy and Error-Free API Management
Bogdan Vlad
Apr 10, 2024
Genezio has Streamlit Support: Simplified AI Prototyping and Interactive Dashboards
Cristi Miloiu
Feb 13, 2025
Getting Started with Django: Build Your First Web Hosted App in 10 Minutes
Cristi Miloiu
Dec 12, 2024